Magento usually has bugs (thank you, Captain Obvious) after the release of a version, that are later corrected on subsequent releases (known issues).
Using patches to change core files in Magento is not a bad practice when the goal behind it is to bring core fixes existing on newer versions to the one we have running, avoiding so a full upgrade.
How to apply a patch
I'm starting the other way around as applying a patch might be more common than actually creating one, specially because Magento itself releases patches for its own platform, third-party vendors release patches for their extensions, and the community also makes patches for others to use.
There's a well known Composer package named cweagans/composer-patches that handles the patches and applies them to the original Magento modules every time you run composer install
and/or composer update
.
Technically speaking, this tool installs all Composer packages required, then removes the Magento modules affected by the patches we are including, and re-install those modules with the code changes applied.
➜ ~ composer install
Gathering patches from patch file.
Removing package magento/module-customer so that it can be re-installed and re-patched.
- Removing magento/module-customer (102.0.5)
Loading composer repositories with package information
Installing dependencies (including require-dev) from lock file
Package operations: 1 install, 0 updates, 0 removals
Gathering patches from patch file.
Gathering patches for dependencies. This might take a minute.
- Installing magento/module-customer (102.0.5): Loading from cache
- Applying patches for magento/module-customer
patches/composer/magento/module-customer/22952.patch (#22952 - Delegated account creation fails with custom attributes present in customer address)
For making this happen, first you need to add your .patch
file in the patches/composer/magento/[module-name]/
folder (relative to the Magento root, at the same level your composer.json
file lives).
The name of the .patch
file doesn't really matter, but I personally like to use the number of the GitHub issue where the known issue was discussed, if that even exists. Otherwise, any descriptive name would do.
For the [module-name]
folder name use the Composer package name containing the files you are patching.
As an example, the Composer package name for the Magento_Customer
module is module-customer
. This information is on the composer.json
file of each package, inside the vendor
folder.
Finally, on the root composer.json
file, inside the extra
node create the patches
node and define the patch to apply as the following example.
"extra": {
"composer-exit-on-patch-failure": true,
"patches": {
"magento/module-customer": {
"#22952 - Delegated account creation fails with custom attributes present in customer address": "patches/composer/magento/module-customer/22952.patch"
}
}
}
See that I'm adding a description to what the patch does, and the GitHub issue number, followed by the relative path to the .patch
file itself.
How to create a patch
Identify the core file you would like to change using a patch.
For the sake of an example, I'm going to patch the vendor/magento/module-customer/Model/Address.php
file to apply a fix for the "Delegated account creation fails with custom attributes present in customer address" issue reported in the magento/magento2 repo itself.
Create an exact copy of the file, and make all the necessary changes.
If you are using PhpStorm, you can create a "scratch file" to hold any code temporary, like a draft, by pressing CMD + Shift + N
.
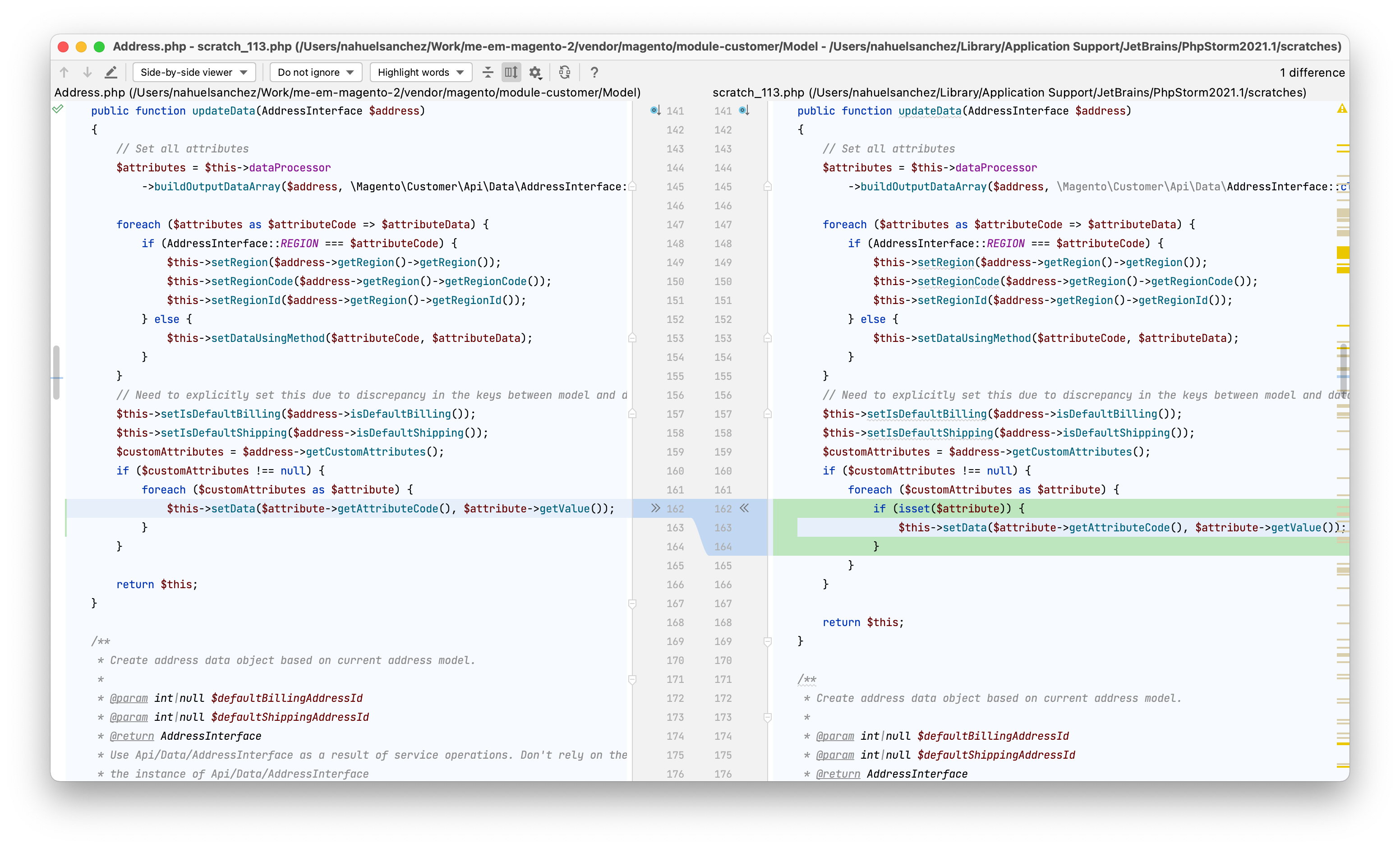
Output the changes into a .patch
file using the diff
command on the Terminal while standing in the root of your Magento.
➜ ~ diff -Naur path/to/old/file.php path/to/new/file.php > example.patch
Following my example, I need to execute the following...
~ diff -Naur vendor/magento/module-customer/Model/Address.php /Users/nahuelsanchez/Library/Application\ Support/JetBrains/PhpStorm2021.1/scratches/scratch_113.php > example.patch
...in order to get my example.patch
file.
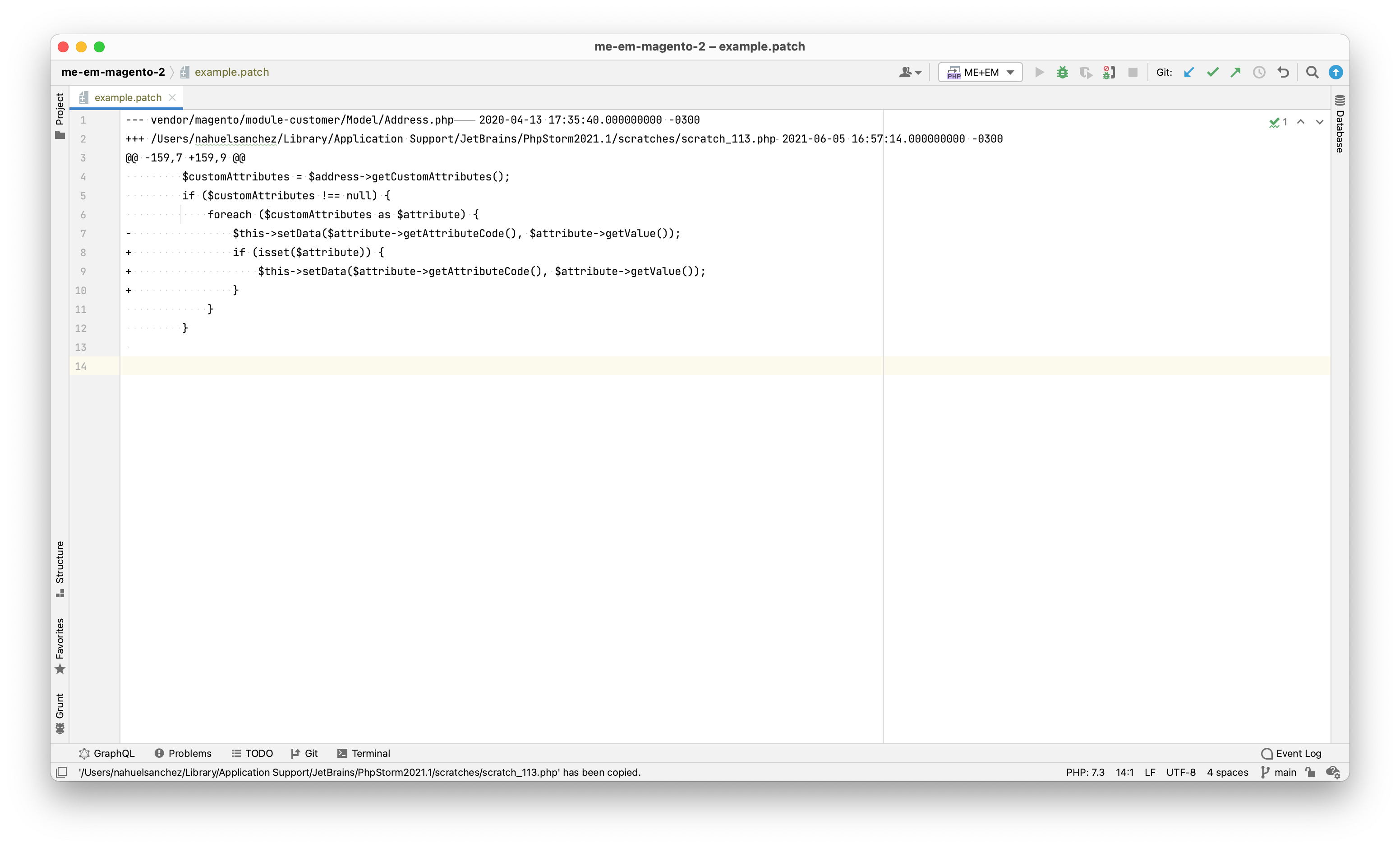
Unfortunately, here's when we need to perform some manual intervention on the generated example.patch
file.
First, remove the date and time appearing the end of the first two lines.
Second, you can see that the first path is the real path to the original file living in the vendor
folder. Add a a/
prefix to it.
Third, replace the second path with the first path, but using the b/
prefix instead.
Fourth, and finally, add a new line on top of everything with diff --git a/vendor/core/file.php b/vendor/core/file.php
.
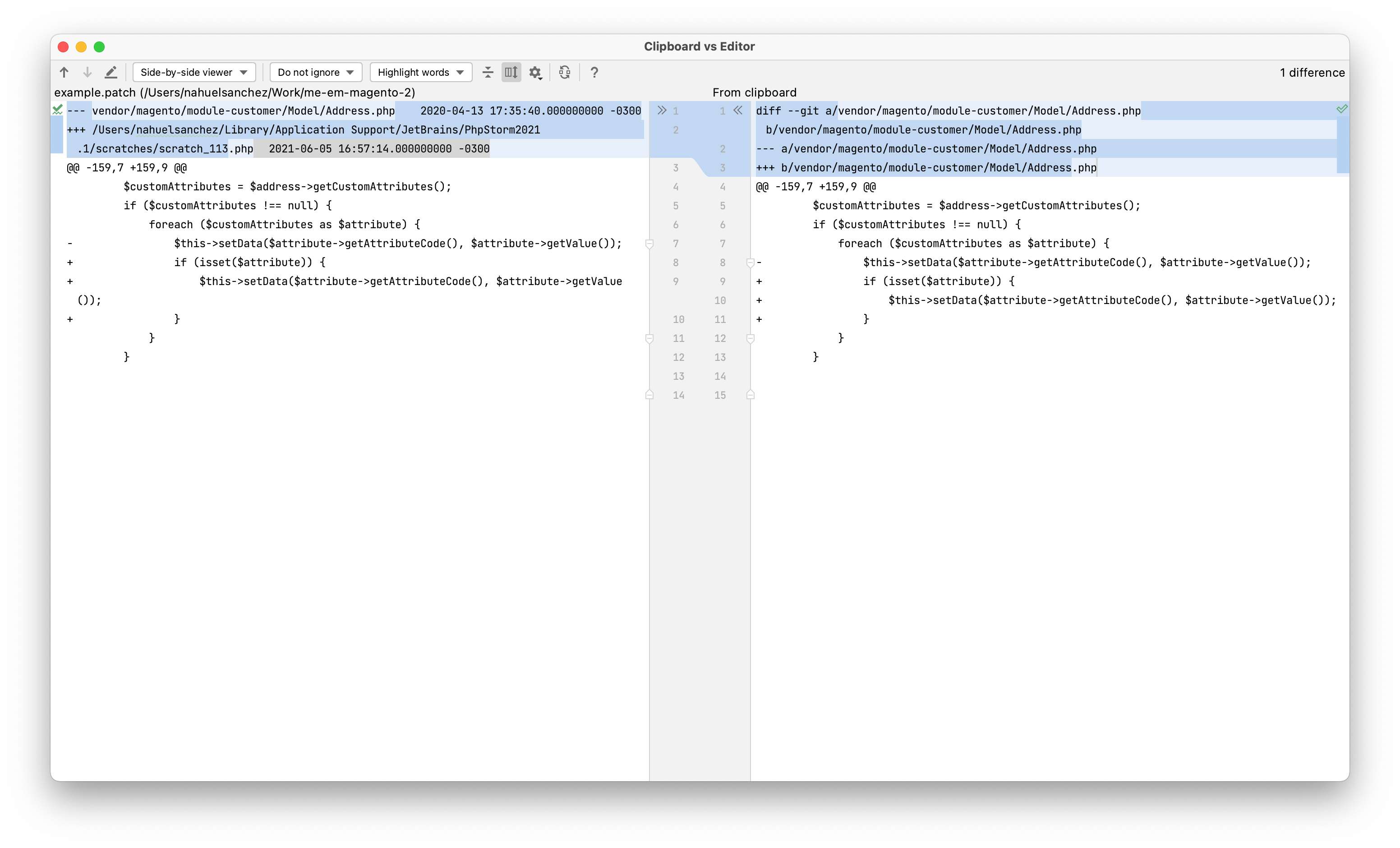
Do not touch anything else there, not even the empty lines at the end (if any).
The resulting file ready to be used as a patch should look as the following example:
diff --git a/vendor/magento/module-customer/Model/Address.php b/vendor/magento/module-customer/Model/Address.php
--- a/vendor/magento/module-customer/Model/Address.php
+++ b/vendor/magento/module-customer/Model/Address.php
@@ -159,7 +159,9 @@
$customAttributes = $address->getCustomAttributes();
if ($customAttributes !== null) {
foreach ($customAttributes as $attribute) {
- $this->setData($attribute->getAttributeCode(), $attribute->getValue());
+ if (isset($attribute)) {
+ $this->setData($attribute->getAttributeCode(), $attribute->getValue());
+ }
}
}